自动生成图集
作者:追风剑情 发布于:2018-1-30 11:04 分类:Unity3d
示例代码
using UnityEngine; using UnityEditor; using System.IO; using System.Collections; using System.Collections.Generic; public class MenuEditor { [MenuItem("Tools/Generate Atlas")] public static void GenerateAtlas() { Object activeObject = Selection.activeObject; string name = activeObject.name; Texture2D texture = new Texture2D(2048, 2048, TextureFormat.RGBA32, false, true); Object[] objs = Selection.GetFiltered(typeof(Texture2D), SelectionMode.DeepAssets); if (null == objs) return; Texture2D[] texArr = new Texture2D[objs.Length]; for (int i = 0; i < objs.Length; i++) { texArr[i] = objs[i] as Texture2D; SetTextureReadable(texArr[i], true); } GameObject go = null; UIAtlas uiatlas = null; go = Resources.LoadAssetAtPath<GameObject>(string.Format("Assets/{0}.prefab", name)); if (null != go) { uiatlas = go.GetComponent<UIAtlas>();//获取已存在的atlas } //注意: PackTextures()返回的是UV坐标,以图片左上角为(0,0)点(从上到下,从左到右),实际生成的texture中的sprite是从从左到右,下到上排列的。 Rect[] rectArr = texture.PackTextures(texArr, 0, 2048); List<UISpriteData> spriteList = new List<UISpriteData>(); for (int i = 0; i < texArr.Length; i++) { Rect rect = rectArr[i]; //sprite在图集中的UV坐标 float uv_x = rect.x; float uv_y = 1 - rect.y + rect.height;//反转Y坐标 float uv_w = rect.width; float uv_h = rect.height; //sprite在图集中的像素坐标 int pixel_x = (int)(uv_x * texture.width); int pixel_y = (int)(uv_y * texture.height); int pixel_w = (int)(uv_w * texture.width); int pixel_h = (int)(uv_h * texture.height); UISpriteData sd = new UISpriteData(); sd.name = texArr[i].name; sd.SetRect(pixel_x, pixel_y, pixel_w, pixel_h); spriteList.Add(sd); if(null != uiatlas){ UISpriteData old_sd = uiatlas.GetSprite(sd.name); if(null != old_sd){ sd.CopyBorderFrom(old_sd);//保留原来设置的九宫缩放信息 } } } Material mat = new Material(Shader.Find("Unlit/Transparent Colored")); go = new GameObject("atlas"); uiatlas = go.AddComponent<UIAtlas>(); uiatlas.spriteList = spriteList; uiatlas.spriteMaterial = mat; uiatlas.MarkSpriteListAsChanged(); //这里的保存路径根据实际情况修改吧 SavePNG(string.Format("Assets/{0}.png", name), texture); AssetDatabase.CreateAsset(mat, string.Format("Assets/{0}.mat", name)); PrefabUtility.CreatePrefab(string.Format("Assets/{0}.prefab", name), go); GameObject.DestroyImmediate(go); GameObject.DestroyImmediate(texture); AssetDatabase.SaveAssets(); AssetDatabase.Refresh(ImportAssetOptions.ForceUpdate); //等上面的资源保存后再设值才生效 mat.mainTexture = Resources.LoadAssetAtPath<Texture>(string.Format("Assets/{0}.png", name)); } static void SetTextureReadable(Texture2D tex, bool readable) { string texPath = AssetDatabase.GetAssetPath(tex); AssetImporter importer = AssetImporter.GetAtPath(texPath); TextureImporter texImporter = importer as TextureImporter; texImporter.textureType = TextureImporterType.Advanced; texImporter.textureFormat = TextureImporterFormat.RGBA32; texImporter.isReadable = readable; AssetDatabase.ImportAsset(texPath); } //保存Texture static void SavePNG(string filePath, Texture2D texture) { if (null == texture) return; try { string dir = Path.GetDirectoryName(filePath); if (!Directory.Exists(dir)) Directory.CreateDirectory(dir); //texture必须为ARGB32,RGBA32,或者是Alpha8且可读,否则这句会报错. byte[] pngData = texture.EncodeToPNG(); File.WriteAllBytes(filePath, pngData); } catch (System.Exception ex) { Debug.Log(ex.StackTrace); } } }
工程截图
标签: Unity3d
日历
最新文章
随机文章
热门文章
分类
存档
- 2024年4月(9)
- 2024年3月(11)
- 2024年2月(24)
- 2024年1月(12)
- 2023年12月(3)
- 2023年11月(9)
- 2023年10月(7)
- 2023年9月(2)
- 2023年8月(7)
- 2023年7月(9)
- 2023年6月(6)
- 2023年5月(7)
- 2023年4月(11)
- 2023年3月(6)
- 2023年2月(11)
- 2023年1月(8)
- 2022年12月(2)
- 2022年11月(4)
- 2022年10月(10)
- 2022年9月(2)
- 2022年8月(13)
- 2022年7月(7)
- 2022年6月(11)
- 2022年5月(18)
- 2022年4月(29)
- 2022年3月(5)
- 2022年2月(6)
- 2022年1月(8)
- 2021年12月(5)
- 2021年11月(3)
- 2021年10月(4)
- 2021年9月(9)
- 2021年8月(14)
- 2021年7月(8)
- 2021年6月(5)
- 2021年5月(2)
- 2021年4月(3)
- 2021年3月(7)
- 2021年2月(2)
- 2021年1月(8)
- 2020年12月(7)
- 2020年11月(2)
- 2020年10月(6)
- 2020年9月(9)
- 2020年8月(10)
- 2020年7月(9)
- 2020年6月(18)
- 2020年5月(4)
- 2020年4月(25)
- 2020年3月(38)
- 2020年1月(21)
- 2019年12月(13)
- 2019年11月(29)
- 2019年10月(44)
- 2019年9月(17)
- 2019年8月(18)
- 2019年7月(25)
- 2019年6月(25)
- 2019年5月(17)
- 2019年4月(10)
- 2019年3月(36)
- 2019年2月(35)
- 2019年1月(28)
- 2018年12月(30)
- 2018年11月(22)
- 2018年10月(4)
- 2018年9月(7)
- 2018年8月(13)
- 2018年7月(13)
- 2018年6月(6)
- 2018年5月(5)
- 2018年4月(13)
- 2018年3月(5)
- 2018年2月(3)
- 2018年1月(8)
- 2017年12月(35)
- 2017年11月(17)
- 2017年10月(16)
- 2017年9月(17)
- 2017年8月(20)
- 2017年7月(34)
- 2017年6月(17)
- 2017年5月(15)
- 2017年4月(32)
- 2017年3月(8)
- 2017年2月(2)
- 2017年1月(5)
- 2016年12月(14)
- 2016年11月(26)
- 2016年10月(12)
- 2016年9月(25)
- 2016年8月(32)
- 2016年7月(14)
- 2016年6月(21)
- 2016年5月(17)
- 2016年4月(13)
- 2016年3月(8)
- 2016年2月(8)
- 2016年1月(18)
- 2015年12月(13)
- 2015年11月(15)
- 2015年10月(12)
- 2015年9月(18)
- 2015年8月(21)
- 2015年7月(35)
- 2015年6月(13)
- 2015年5月(9)
- 2015年4月(4)
- 2015年3月(5)
- 2015年2月(4)
- 2015年1月(13)
- 2014年12月(7)
- 2014年11月(5)
- 2014年10月(4)
- 2014年9月(8)
- 2014年8月(16)
- 2014年7月(26)
- 2014年6月(22)
- 2014年5月(28)
- 2014年4月(15)
友情链接
- Unity官网
- Unity圣典
- Unity在线手册
- Unity中文手册(圣典)
- Unity官方中文论坛
- Unity游戏蛮牛用户文档
- Unity下载存档
- Unity引擎源码下载
- Unity服务
- Unity Ads
- wiki.unity3d
- Visual Studio Code官网
- SenseAR开发文档
- MSDN
- C# 参考
- C# 编程指南
- .NET Framework类库
- .NET 文档
- .NET 开发
- WPF官方文档
- uLua
- xLua
- SharpZipLib
- Protobuf-net
- Protobuf.js
- OpenSSL
- OPEN CASCADE
- JSON
- MessagePack
- C在线工具
- 游戏蛮牛
- GreenVPN
- 聚合数据
- 热云
- 融云
- 腾讯云
- 腾讯开放平台
- 腾讯游戏服务
- 腾讯游戏开发者平台
- 腾讯课堂
- 微信开放平台
- 腾讯实时音视频
- 腾讯即时通信IM
- 微信公众平台技术文档
- 白鹭引擎官网
- 白鹭引擎开放平台
- 白鹭引擎开发文档
- FairyGUI编辑器
- PureMVC-TypeScript
- 讯飞开放平台
- 亲加通讯云
- Cygwin
- Mono开发者联盟
- Scut游戏服务器引擎
- KBEngine游戏服务器引擎
- Photon游戏服务器引擎
- 码云
- SharpSvn
- 腾讯bugly
- 4399原创平台
- 开源中国
- Firebase
- Firebase-Admob-Unity
- google-services-unity
- Firebase SDK for Unity
- Google-Firebase-SDK
- AppsFlyer SDK
- android-repository
- CQASO
- Facebook开发者平台
- gradle下载
- GradleBuildTool下载
- Android Developers
- Google中国开发者
- AndroidDevTools
- Android社区
- Android开发工具
- Google Play Games Services
- Google商店
- Google APIs for Android
- 金钱豹VPN
- TouchSense SDK
- MakeHuman
- Online RSA Key Converter
- Windows UWP应用
- Visual Studio For Unity
- Open CASCADE Technology
- 慕课网
- 阿里云服务器ECS
- 在线免费文字转语音系统
- AI Studio
- 网云穿
- 百度网盘开放平台
- 迅捷画图
- 菜鸟工具
- [CSDN] 程序员研修院
- 华为人脸识别
- 百度AR导航导览SDK
- 海康威视官网
- 海康开放平台
- 海康SDK下载
交流QQ群
-
Flash游戏设计: 86184192
Unity游戏设计: 171855449
游戏设计订阅号
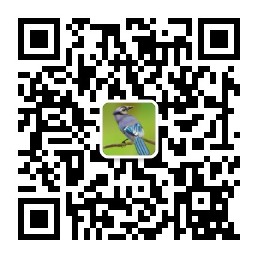