MRTK-Solver
作者:追风剑情 发布于:2023-7-5 18:50 分类:Unity3d
所有解算器要从中派生的抽象基类。参见 求解器概述 - MRTK2
using System; using UnityEngine; using UnityEngine.Serialization; namespace Microsoft.MixedReality.Toolkit.Utilities.Solvers { [RequireComponent(typeof(SolverHandler))] public abstract class Solver : MonoBehaviour { //如果为true,将计算位置和方向,但不应用于其他组件。 private bool updateLinkedTransform = false; //移动插值时间,值越大移动越缓慢 //参见:UpdateWorkingPositionToGoal() private float moveLerpTime = 0.1f; //旋转插值时间,值越大旋转越缓慢 //参见:UpdateWorkingRotationToGoal() private float rotateLerpTime = 0.1f; //缩放插值时间,值越大缩放越缓慢 //参见:UpdateWorkingScaleToGoal() private float scaleLerpTime = 0; //true:初始化时保持transform的localScale值不变。 //参见 Awake() private bool maintainScaleOnInitialization = true; //是否采用平滑插值。 //参见: UpdateWorkingPositionToGoal()、UpdateWorkingRotationToGoal()、UpdateWorkingScaleToGoal() private bool smoothing = true; //解算器生命周期,0:无限 //参见: SolverUpdateEntry() private float lifetime = 0; //参见: Awake() protected SolverHandler SolverHandler; /// <summary> /// 最终坐标,由GameObject上所有解算器共同计算所得。 /// </summary> protected Vector3 GoalPosition { get { return SolverHandler.GoalPosition; } set { SolverHandler.GoalPosition = value; } } /// <summary> /// 最终旋转,由GameObject上所有解算器共同计算所得。 /// </summary> protected Quaternion GoalRotation { get { return SolverHandler.GoalRotation; } set { SolverHandler.GoalRotation = value; } } /// <summary> /// 最终缩放,由GameObject上所有解算器共同计算所得。 /// </summary> protected Vector3 GoalScale { get { return SolverHandler.GoalScale; } set { SolverHandler.GoalScale = value; } } /// <summary> /// 当前坐标 /// </summary> public Vector3 WorkingPosition { get { return updateLinkedTransform ? GoalPosition : transform.position; } protected set { if (updateLinkedTransform) { GoalPosition = value; } else { transform.position = value; } } } /// <summary> /// 当前旋转 /// </summary> public Quaternion WorkingRotation { get { return updateLinkedTransform ? GoalRotation : transform.rotation; } protected set { if (updateLinkedTransform) { GoalRotation = value; } else { transform.rotation = value; } } } /// <summary> /// 当前缩放 /// </summary> public Vector3 WorkingScale { get { return updateLinkedTransform ? GoalScale : transform.localScale; } protected set { if (updateLinkedTransform) { GoalScale = value; } else { transform.localScale = value; } } } #region MonoBehaviour Implementation protected virtual void Awake() { if (SolverHandler == null) { SolverHandler = GetComponent<SolverHandler>(); } if (updateLinkedTransform && SolverHandler == null) { Debug.LogError("No SolverHandler component found on " + name + " when UpdateLinkedTransform was set to true! Disabling UpdateLinkedTransform."); updateLinkedTransform = false; } GoalScale = maintainScaleOnInitialization ? transform.localScale : Vector3.one; } protected virtual void OnEnable() { if (SolverHandler != null) { SnapGoalTo(GoalPosition, GoalRotation, GoalScale); } currentLifetime = 0; } protected virtual void Start() { if (SolverHandler != null) { //注册解算器 SolverHandler.RegisterSolver(this); } } protected virtual void OnDestroy() { if (SolverHandler != null) { //注销解算器 SolverHandler.UnregisterSolver(this); } } #endregion MonoBehaviour Implementation /// <summary> /// 由子类实现,更新游戏内容变换。 /// </summary> public abstract void SolverUpdate(); /// <summary> /// 此方法在SolverHandler类的LateUpdate()中被每帧调用。 /// </summary> public void SolverUpdateEntry() { currentLifetime += SolverHandler.DeltaTime; //判断解算器的生命周期是否结束 if (lifetime > 0 && currentLifetime >= lifetime) { enabled = false; return; } SolverUpdate(); UpdateWorkingToGoal(); } /// <summary> /// 更新解算器的变换值 /// </summary> public virtual void SnapTo(Vector3 position, Quaternion rotation, Vector3 scale) { SnapGoalTo(position, rotation, scale); WorkingPosition = position; WorkingRotation = rotation; WorkingScale = scale; } /// <summary> /// 更新解算器的最终变换值 /// </summary> public virtual void SnapGoalTo(Vector3 position, Quaternion rotation, Vector3 scale) { GoalPosition = position; GoalRotation = rotation; GoalScale = scale; } /// <summary> /// 坐标偏移 /// </summary> public virtual void AddOffset(Vector3 offset) { GoalPosition += offset; } /// <summary> /// 更新Transform /// </summary> protected void UpdateTransformToGoal() { if (smoothing) { Vector3 pos = transform.position; Quaternion rot = transform.rotation; Vector3 scale = transform.localScale; pos = SmoothTo(pos, GoalPosition, SolverHandler.DeltaTime, moveLerpTime); rot = SmoothTo(rot, GoalRotation, SolverHandler.DeltaTime, rotateLerpTime); scale = SmoothTo(scale, GoalScale, SolverHandler.DeltaTime, scaleLerpTime); transform.position = pos; transform.rotation = rot; transform.localScale = scale; } else { transform.position = GoalPosition; transform.rotation = GoalRotation; transform.localScale = GoalScale; } } public void UpdateWorkingToGoal() { UpdateWorkingPositionToGoal(); UpdateWorkingRotationToGoal(); UpdateWorkingScaleToGoal(); } public void UpdateWorkingPositionToGoal() { WorkingPosition = smoothing ? SmoothTo(WorkingPosition, GoalPosition, SolverHandler.DeltaTime, moveLerpTime) : GoalPosition; } public void UpdateWorkingRotationToGoal() { WorkingRotation = smoothing ? SmoothTo(WorkingRotation, GoalRotation, SolverHandler.DeltaTime, rotateLerpTime) : GoalRotation; } public void UpdateWorkingScaleToGoal() { WorkingScale = smoothing ? SmoothTo(WorkingScale, GoalScale, SolverHandler.DeltaTime, scaleLerpTime) : GoalScale; } // 向量平滑插值 public static Vector3 SmoothTo(Vector3 source, Vector3 goal, float deltaTime, float lerpTime) { return Vector3.Lerp(source, goal, lerpTime.Equals(0.0f) ? 1f : deltaTime / lerpTime); } // 四元数平滑插值 public static Quaternion SmoothTo(Quaternion source, Quaternion goal, float deltaTime, float lerpTime) { return Quaternion.Slerp(source, goal, lerpTime.Equals(0.0f) ? 1f : deltaTime / lerpTime); } } }
标签: Unity3d
« MRTK-HandConstraint
|
平面»
日历
最新文章
随机文章
热门文章
分类
存档
- 2024年4月(9)
- 2024年3月(11)
- 2024年2月(24)
- 2024年1月(12)
- 2023年12月(3)
- 2023年11月(9)
- 2023年10月(7)
- 2023年9月(2)
- 2023年8月(7)
- 2023年7月(9)
- 2023年6月(6)
- 2023年5月(7)
- 2023年4月(11)
- 2023年3月(6)
- 2023年2月(11)
- 2023年1月(8)
- 2022年12月(2)
- 2022年11月(4)
- 2022年10月(10)
- 2022年9月(2)
- 2022年8月(13)
- 2022年7月(7)
- 2022年6月(11)
- 2022年5月(18)
- 2022年4月(29)
- 2022年3月(5)
- 2022年2月(6)
- 2022年1月(8)
- 2021年12月(5)
- 2021年11月(3)
- 2021年10月(4)
- 2021年9月(9)
- 2021年8月(14)
- 2021年7月(8)
- 2021年6月(5)
- 2021年5月(2)
- 2021年4月(3)
- 2021年3月(7)
- 2021年2月(2)
- 2021年1月(8)
- 2020年12月(7)
- 2020年11月(2)
- 2020年10月(6)
- 2020年9月(9)
- 2020年8月(10)
- 2020年7月(9)
- 2020年6月(18)
- 2020年5月(4)
- 2020年4月(25)
- 2020年3月(38)
- 2020年1月(21)
- 2019年12月(13)
- 2019年11月(29)
- 2019年10月(44)
- 2019年9月(17)
- 2019年8月(18)
- 2019年7月(25)
- 2019年6月(25)
- 2019年5月(17)
- 2019年4月(10)
- 2019年3月(36)
- 2019年2月(35)
- 2019年1月(28)
- 2018年12月(30)
- 2018年11月(22)
- 2018年10月(4)
- 2018年9月(7)
- 2018年8月(13)
- 2018年7月(13)
- 2018年6月(6)
- 2018年5月(5)
- 2018年4月(13)
- 2018年3月(5)
- 2018年2月(3)
- 2018年1月(8)
- 2017年12月(35)
- 2017年11月(17)
- 2017年10月(16)
- 2017年9月(17)
- 2017年8月(20)
- 2017年7月(34)
- 2017年6月(17)
- 2017年5月(15)
- 2017年4月(32)
- 2017年3月(8)
- 2017年2月(2)
- 2017年1月(5)
- 2016年12月(14)
- 2016年11月(26)
- 2016年10月(12)
- 2016年9月(25)
- 2016年8月(32)
- 2016年7月(14)
- 2016年6月(21)
- 2016年5月(17)
- 2016年4月(13)
- 2016年3月(8)
- 2016年2月(8)
- 2016年1月(18)
- 2015年12月(13)
- 2015年11月(15)
- 2015年10月(12)
- 2015年9月(18)
- 2015年8月(21)
- 2015年7月(35)
- 2015年6月(13)
- 2015年5月(9)
- 2015年4月(4)
- 2015年3月(5)
- 2015年2月(4)
- 2015年1月(13)
- 2014年12月(7)
- 2014年11月(5)
- 2014年10月(4)
- 2014年9月(8)
- 2014年8月(16)
- 2014年7月(26)
- 2014年6月(22)
- 2014年5月(28)
- 2014年4月(15)
友情链接
- Unity官网
- Unity圣典
- Unity在线手册
- Unity中文手册(圣典)
- Unity官方中文论坛
- Unity游戏蛮牛用户文档
- Unity下载存档
- Unity引擎源码下载
- Unity服务
- Unity Ads
- wiki.unity3d
- Visual Studio Code官网
- SenseAR开发文档
- MSDN
- C# 参考
- C# 编程指南
- .NET Framework类库
- .NET 文档
- .NET 开发
- WPF官方文档
- uLua
- xLua
- SharpZipLib
- Protobuf-net
- Protobuf.js
- OpenSSL
- OPEN CASCADE
- JSON
- MessagePack
- C在线工具
- 游戏蛮牛
- GreenVPN
- 聚合数据
- 热云
- 融云
- 腾讯云
- 腾讯开放平台
- 腾讯游戏服务
- 腾讯游戏开发者平台
- 腾讯课堂
- 微信开放平台
- 腾讯实时音视频
- 腾讯即时通信IM
- 微信公众平台技术文档
- 白鹭引擎官网
- 白鹭引擎开放平台
- 白鹭引擎开发文档
- FairyGUI编辑器
- PureMVC-TypeScript
- 讯飞开放平台
- 亲加通讯云
- Cygwin
- Mono开发者联盟
- Scut游戏服务器引擎
- KBEngine游戏服务器引擎
- Photon游戏服务器引擎
- 码云
- SharpSvn
- 腾讯bugly
- 4399原创平台
- 开源中国
- Firebase
- Firebase-Admob-Unity
- google-services-unity
- Firebase SDK for Unity
- Google-Firebase-SDK
- AppsFlyer SDK
- android-repository
- CQASO
- Facebook开发者平台
- gradle下载
- GradleBuildTool下载
- Android Developers
- Google中国开发者
- AndroidDevTools
- Android社区
- Android开发工具
- Google Play Games Services
- Google商店
- Google APIs for Android
- 金钱豹VPN
- TouchSense SDK
- MakeHuman
- Online RSA Key Converter
- Windows UWP应用
- Visual Studio For Unity
- Open CASCADE Technology
- 慕课网
- 阿里云服务器ECS
- 在线免费文字转语音系统
- AI Studio
- 网云穿
- 百度网盘开放平台
- 迅捷画图
- 菜鸟工具
- [CSDN] 程序员研修院
- 华为人脸识别
- 百度AR导航导览SDK
- 海康威视官网
- 海康开放平台
- 海康SDK下载
交流QQ群
-
Flash游戏设计: 86184192
Unity游戏设计: 171855449
游戏设计订阅号
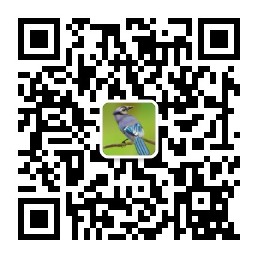