Hololens 拍照
作者:追风剑情 发布于:2023-4-20 19:03 分类:Unity3d
using System; using System.Collections; using System.Linq; using System.IO; #if WINDOWS_UWP using Windows.Storage; #endif using UnityEngine; using UnityEngine.UI; using UnityEngine.Windows.WebCam; /// <summary> /// 拍照 /// </summary> public class WebCamPhoto : MonoBehaviour { public bool showHolograms = false; [SerializeField] private RawImage photoRawImage; [SerializeField] private Text debugText; private Resolution resolution; private PhotoCapture photoCapture; private Texture2D photoTexture2D; //拍照成功事件 public Action<Texture2D> OnPhotoCompleted; //拍照出错事件 public Action OnPhotoError; private void Start() { //Debug.Log("HoloLens 2 支持的拍照分辨率有:"); //HoloLens 2支持的拍照分辨率为: 3904 x 2196 //获取设备支持的拍照分辨率 //foreach (Resolution res in PhotoCapture.SupportedResolutions) //{ // Debug.LogFormat("Resolution: width={0}, height={1}", res.width, res.height); //} //返回最大分辨率 resolution = PhotoCapture.SupportedResolutions.OrderByDescending((res) => res.width * res.height).First(); } private void Log(string s) { Debug.Log(s); if (debugText != null) debugText.text = s; } private void LogFormat(string format, params object[] args) { Debug.LogFormat(format, args); if (debugText != null) debugText.text = string.Format(format, args); } // 拍照 public void TakePhoto() { if (photoCapture != null) photoCapture.TakePhotoAsync(OnCapturedToMemory); else StartCoroutine(StartCameraCapture()); } // 启动摄像头 private IEnumerator StartCameraCapture() { //检测权限 if (!Application.HasUserAuthorization(UserAuthorization.WebCam)) { yield return Application.RequestUserAuthorization(UserAuthorization.WebCam); } if (Application.HasUserAuthorization(UserAuthorization.WebCam)) { //第1个参数 true:照片包含全息画面,false:仅硬件摄像头画面 PhotoCapture.CreateAsync(showHolograms, OnCaptureResourceCreated); } } // 创建PhotoCapture对象回调 private void OnCaptureResourceCreated(PhotoCapture captureObject) { photoCapture = captureObject; //设置拍照参数 CameraParameters setupParams = new CameraParameters(WebCamMode.PhotoMode); setupParams.hologramOpacity = 0.0f; setupParams.cameraResolutionWidth = resolution.width; setupParams.cameraResolutionHeight = resolution.height; setupParams.pixelFormat = CapturePixelFormat.BGRA32; //开始启动摄像头 photoCapture.StartPhotoModeAsync(setupParams, OnPhotoModeStarted); } // 摄像头启动回调 private void OnPhotoModeStarted(PhotoCapture.PhotoCaptureResult result) { if (!result.success) { Log("启动拍照模式失败"); OnPhotoError?.Invoke(); return; } //执行拍照 photoCapture.TakePhotoAsync(OnCapturedToMemory); } // 拍照完成回调 private void OnCapturedToMemory(PhotoCapture.PhotoCaptureResult result, PhotoCaptureFrame photoCaptureFrame) { if (!result.success) { Log("不能保存照片到内存"); OnPhotoError?.Invoke(); return; } //利用照片数据创建新的Texture2D //List<byte> byteBuffer = new List<byte>(); //setupParams.pixelFormat设置的是什么图片格式,CopyRawImageDataIntoBuffer()方法读出的就是对应格式的图片数据 //photoCaptureFrame.CopyRawImageDataIntoBuffer(byteBuffer); //photoTexture2D = new Texture2D(resolution.width, resolution.height, TextureFormat.BGRA32, false); //tex.LoadRawTextureData(byteBuffer.ToArray()); //tex.Apply(); //直接将照片数据赋值给Texture2D photoTexture2D = new Texture2D(resolution.width, resolution.height, TextureFormat.BGRA32, false); photoCaptureFrame.UploadImageDataToTexture(photoTexture2D); if (photoRawImage != null) photoRawImage.texture = photoTexture2D; //释放内存中的照片数据 photoCaptureFrame.Dispose(); //停止摄像头 photoCapture.StopPhotoModeAsync(OnPhotoModeStopped); } // 停止摄像头回调 private void OnPhotoModeStopped(PhotoCapture.PhotoCaptureResult result) { if (result.success) { //释放拍照占用的资源 photoCapture.Dispose(); photoCapture = null; } Log("拍照完成"); //SavePhoto(); //拍照完成事件 OnPhotoCompleted?.Invoke(photoTexture2D); } // 保存照片到 Camera Roll 文件夹 private void SavePhoto() { string fileName = "photo.jpg"; string filePath = Path.Combine(Application.persistentDataPath, fileName); byte[] bytes = photoTexture2D.EncodeToJPG(); File.WriteAllBytes(filePath, bytes); #if WINDOWS_UWP //注意:需要勾选上图片库(PicturesLibrary)设备功能,才能访问CameraRoll文件夹 //注意:无法直接保存到CameraRoll文件夹 //注意:HoloLens 2 移动照片时,全息影响会闪烁一下,原因不明 var cameraRollFolder = Windows.Storage.KnownFolders.CameraRoll.Path; File.Move(filePath, Path.Combine(cameraRollFolder, fileName)); #endif } }
标签: Unity3d
日历
最新文章
随机文章
热门文章
分类
存档
- 2024年7月(11)
- 2024年6月(3)
- 2024年5月(9)
- 2024年4月(10)
- 2024年3月(11)
- 2024年2月(24)
- 2024年1月(12)
- 2023年12月(3)
- 2023年11月(9)
- 2023年10月(7)
- 2023年9月(2)
- 2023年8月(7)
- 2023年7月(9)
- 2023年6月(6)
- 2023年5月(7)
- 2023年4月(11)
- 2023年3月(6)
- 2023年2月(11)
- 2023年1月(8)
- 2022年12月(2)
- 2022年11月(4)
- 2022年10月(10)
- 2022年9月(2)
- 2022年8月(13)
- 2022年7月(7)
- 2022年6月(11)
- 2022年5月(18)
- 2022年4月(29)
- 2022年3月(5)
- 2022年2月(6)
- 2022年1月(8)
- 2021年12月(5)
- 2021年11月(3)
- 2021年10月(4)
- 2021年9月(9)
- 2021年8月(14)
- 2021年7月(8)
- 2021年6月(5)
- 2021年5月(2)
- 2021年4月(3)
- 2021年3月(7)
- 2021年2月(2)
- 2021年1月(8)
- 2020年12月(7)
- 2020年11月(2)
- 2020年10月(6)
- 2020年9月(9)
- 2020年8月(10)
- 2020年7月(9)
- 2020年6月(18)
- 2020年5月(4)
- 2020年4月(25)
- 2020年3月(38)
- 2020年1月(21)
- 2019年12月(13)
- 2019年11月(29)
- 2019年10月(44)
- 2019年9月(17)
- 2019年8月(18)
- 2019年7月(25)
- 2019年6月(25)
- 2019年5月(17)
- 2019年4月(10)
- 2019年3月(36)
- 2019年2月(35)
- 2019年1月(28)
- 2018年12月(30)
- 2018年11月(22)
- 2018年10月(4)
- 2018年9月(7)
- 2018年8月(13)
- 2018年7月(13)
- 2018年6月(6)
- 2018年5月(5)
- 2018年4月(13)
- 2018年3月(5)
- 2018年2月(3)
- 2018年1月(8)
- 2017年12月(35)
- 2017年11月(17)
- 2017年10月(16)
- 2017年9月(17)
- 2017年8月(20)
- 2017年7月(34)
- 2017年6月(17)
- 2017年5月(15)
- 2017年4月(32)
- 2017年3月(8)
- 2017年2月(2)
- 2017年1月(5)
- 2016年12月(14)
- 2016年11月(26)
- 2016年10月(12)
- 2016年9月(25)
- 2016年8月(32)
- 2016年7月(14)
- 2016年6月(21)
- 2016年5月(17)
- 2016年4月(13)
- 2016年3月(8)
- 2016年2月(8)
- 2016年1月(18)
- 2015年12月(13)
- 2015年11月(15)
- 2015年10月(12)
- 2015年9月(18)
- 2015年8月(21)
- 2015年7月(35)
- 2015年6月(13)
- 2015年5月(9)
- 2015年4月(4)
- 2015年3月(5)
- 2015年2月(4)
- 2015年1月(13)
- 2014年12月(7)
- 2014年11月(5)
- 2014年10月(4)
- 2014年9月(8)
- 2014年8月(16)
- 2014年7月(26)
- 2014年6月(22)
- 2014年5月(28)
- 2014年4月(15)
友情链接
- Unity官网
- Unity圣典
- Unity在线手册
- Unity中文手册(圣典)
- Unity官方中文论坛
- Unity游戏蛮牛用户文档
- Unity下载存档
- Unity引擎源码下载
- Unity服务
- Unity Ads
- wiki.unity3d
- Visual Studio Code官网
- SenseAR开发文档
- MSDN
- C# 参考
- C# 编程指南
- .NET Framework类库
- .NET 文档
- .NET 开发
- WPF官方文档
- uLua
- xLua
- SharpZipLib
- Protobuf-net
- Protobuf.js
- OpenSSL
- OPEN CASCADE
- JSON
- MessagePack
- C在线工具
- 游戏蛮牛
- GreenVPN
- 聚合数据
- 热云
- 融云
- 腾讯云
- 腾讯开放平台
- 腾讯游戏服务
- 腾讯游戏开发者平台
- 腾讯课堂
- 微信开放平台
- 腾讯实时音视频
- 腾讯即时通信IM
- 微信公众平台技术文档
- 白鹭引擎官网
- 白鹭引擎开放平台
- 白鹭引擎开发文档
- FairyGUI编辑器
- PureMVC-TypeScript
- 讯飞开放平台
- 亲加通讯云
- Cygwin
- Mono开发者联盟
- Scut游戏服务器引擎
- KBEngine游戏服务器引擎
- Photon游戏服务器引擎
- 码云
- SharpSvn
- 腾讯bugly
- 4399原创平台
- 开源中国
- Firebase
- Firebase-Admob-Unity
- google-services-unity
- Firebase SDK for Unity
- Google-Firebase-SDK
- AppsFlyer SDK
- android-repository
- CQASO
- Facebook开发者平台
- gradle下载
- GradleBuildTool下载
- Android Developers
- Google中国开发者
- AndroidDevTools
- Android社区
- Android开发工具
- Google Play Games Services
- Google商店
- Google APIs for Android
- 金钱豹VPN
- TouchSense SDK
- MakeHuman
- Online RSA Key Converter
- Windows UWP应用
- Visual Studio For Unity
- Open CASCADE Technology
- 慕课网
- 阿里云服务器ECS
- 在线免费文字转语音系统
- AI Studio
- 网云穿
- 百度网盘开放平台
- 迅捷画图
- 菜鸟工具
- [CSDN] 程序员研修院
- 华为人脸识别
- 百度AR导航导览SDK
- 海康威视官网
- 海康开放平台
- 海康SDK下载
交流QQ群
-
Flash游戏设计: 86184192
Unity游戏设计: 171855449
游戏设计订阅号
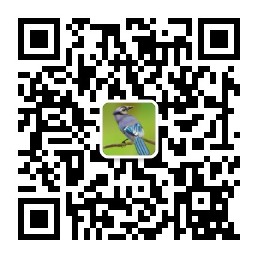