UGUI—跑马灯文本
作者:追风剑情 发布于:2020-4-9 17:03 分类:Unity3d
工程截图
using System; using System.Collections; using System.Collections.Generic; using System.Text.RegularExpressions; using UnityEngine; using UnityEngine.UI; using UnityEngine.Events; using UnityEngine.EventSystems; using UnityEngine.Serialization; /// <summary> /// 滚动文本 (跑马灯效果) /// </summary> public class UIMarqueeText : MonoBehaviour, IPointerClickHandler { [SerializeField] private GameObject root; [SerializeField] private RectTransform m_Mask; [SerializeField] private Text m_Text; [NonSerialized] private RectTransform m_Rect; [SerializeField] private float m_Speed = -30f; [SerializeField] private bool m_Loop = true; //消息列表 [SerializeField] private List<string> m_MessageList = new List<string>(); private float m_MaskWidth, m_TextWidth; private bool m_Preferred = false; private bool m_Playing = false; private bool m_Stared = false; //超链接列表 private List<HyperLink> m_HyperLinkList = new List<HyperLink>(); //超链接点击事件 public Action<HyperLink> OnClickHyperLinkEvent; public struct HyperLink { //区域 public Rect rect; //超链接参数 public string href; //超链接文本 public string text; } private RectTransform rectTransform { get { if (m_Rect == null) m_Rect = GetComponent<RectTransform>(); return m_Rect; } } private float X { get { return rectTransform.anchoredPosition.x; } set { UGUITool.SetAnchoredPositionX(rectTransform, value); } } private void Awake() { if (m_Text == null) m_Text = GetComponent<Text>(); if (m_Mask == null) m_Mask = this.rectTransform.parent.GetComponent<RectTransform>(); } void Start() { m_Stared = true; m_MaskWidth = m_Mask.rect.width; PlayNext(); } void Update() { if (!m_Playing || !m_Preferred) return; float deltaX = m_Speed * Time.deltaTime; //坐标值过小会出现文字抖动,通过Mathf.FloorToInt()去掉小数点后面的值 UGUITool.SetAnchoredPositionOffsetX(rectTransform, Mathf.FloorToInt(deltaX)); // 判断文本是否已跑到最左边 if (X < -(m_MaskWidth + m_TextWidth)) { X = 0; if (m_Loop) //循环播放当前消息 { return; } else { m_Preferred = false; //播放下一条消息 if (m_MessageList.Count > 0) { string msg = m_MessageList[0]; m_MessageList.RemoveAt(0); m_Text.text = msg; StartCoroutine(TextPreferredSize(m_Text)); } else { m_Playing = false; //关闭公告 Hide(); } } } } // 点击事件 public void OnPointerClick(PointerEventData eventData) { Vector2 position = eventData.position; Vector2 rect_position = UGUITool.ScreenPointToLocal(rectTransform, position); HyperLink hyper = GetHyperLinkByPosition(rect_position); if (!string.IsNullOrEmpty(hyper.text)) { //Debug.LogFormat("Click: href={0}, text={1}", hyper.href, hyper.text); if (OnClickHyperLinkEvent != null) OnClickHyperLinkEvent(hyper); } } // 通过点击坐标获取超链接 private HyperLink GetHyperLinkByPosition(Vector2 rect_position) { HyperLink hyperLink = default(HyperLink); float x = rect_position.x; float y = rect_position.y; for (int i = 0; i < m_HyperLinkList.Count; i++) { HyperLink hyper = m_HyperLinkList[i]; if (x > hyper.rect.xMin && x < hyper.rect.xMax) { hyperLink = hyper; break; } } return hyperLink; } // 使Anchors的宽高与Text内容一致 private IEnumerator TextPreferredSize(Text t) { yield return new WaitForEndOfFrame(); TextGenerator tg = t.cachedTextGeneratorForLayout; TextGenerationSettings settings = t.GetGenerationSettings(Vector2.zero); float size = tg.GetPreferredWidth(t.text, settings) / t.pixelsPerUnit; rectTransform.SetSizeWithCurrentAnchors(RectTransform.Axis.Horizontal, size); m_TextWidth = size; m_Preferred = true; yield return new WaitForSeconds(1);//确保TextGenerator生效 ParseHyperLink(t); } // 解析文本中的超链接 private void ParseHyperLink(Text t) { m_HyperLinkList.Clear(); string input = t.text; string nosymbol_text = StripSymbol(input); string pattern = "<a href=\"(?<url>[\\s\\S]*?)\">(?<text>[\\s\\S]*?)</a>"; Regex reg = new Regex(pattern); foreach (Match match in Regex.Matches(input, pattern)) { string url = match.Groups["url"].Value; string text = match.Groups["text"].Value; Capture capture = match.Groups["text"]; //Debug.LogFormat("url={0}; text={1}", url, text); TextGenerator tg = m_Text.cachedTextGeneratorForLayout; //UGUI中标记符的字符不占用文本宽度(即,UICharInfo.charWidth=0) UICharInfo[] charInfos = tg.GetCharactersArray();//数组中包含标记符 int start_index = capture.Index; int end_index = capture.Index + capture.Length; UICharInfo start_info = charInfos[start_index]; UICharInfo end_info = charInfos[end_index]; Rect rect = new Rect(); rect.xMin = start_info.cursorPos.x; rect.xMax = end_info.cursorPos.x; HyperLink hyper = new HyperLink(); hyper.href = url; hyper.text = StripSymbol(text); hyper.rect = rect; m_HyperLinkList.Add(hyper); } } // 返回不含标记符的文本 private string StripSymbol(string text) { string str = string.Empty; List<char> list = new List<char>(); bool findSymbol = false; for (int i=0; i<text.Length; i++) { if (text[i] == '<') { findSymbol = true; continue; } if (text[i] == '>') { findSymbol = false; continue; } if (findSymbol) continue; list.Add(text[i]); } str = new string(list.ToArray()); return str; } private void Hide() { root.SetActive(false); } private void Show() { root.SetActive(true); } // 播放下一条消息 private void PlayNext() { if (m_MessageList.Count <= 0) return; X = 0; string msg = m_MessageList[0]; m_MessageList.RemoveAt(0); m_Text.text = msg; m_Preferred = false; m_Playing = true; StartCoroutine(TextPreferredSize(m_Text)); } // 添加消息 public void AddMessage(string msg) { m_MessageList.Add(msg); if (m_Playing) return; Show(); if (m_Stared) PlayNext(); } }
用到的其他脚本
UGUITool
标签: Unity3d
« 示例:反转后几位
|
示例:十进制转二进制»
日历
最新文章
随机文章
热门文章
分类
存档
- 2024年4月(9)
- 2024年3月(11)
- 2024年2月(24)
- 2024年1月(12)
- 2023年12月(3)
- 2023年11月(9)
- 2023年10月(7)
- 2023年9月(2)
- 2023年8月(7)
- 2023年7月(9)
- 2023年6月(6)
- 2023年5月(7)
- 2023年4月(11)
- 2023年3月(6)
- 2023年2月(11)
- 2023年1月(8)
- 2022年12月(2)
- 2022年11月(4)
- 2022年10月(10)
- 2022年9月(2)
- 2022年8月(13)
- 2022年7月(7)
- 2022年6月(11)
- 2022年5月(18)
- 2022年4月(29)
- 2022年3月(5)
- 2022年2月(6)
- 2022年1月(8)
- 2021年12月(5)
- 2021年11月(3)
- 2021年10月(4)
- 2021年9月(9)
- 2021年8月(14)
- 2021年7月(8)
- 2021年6月(5)
- 2021年5月(2)
- 2021年4月(3)
- 2021年3月(7)
- 2021年2月(2)
- 2021年1月(8)
- 2020年12月(7)
- 2020年11月(2)
- 2020年10月(6)
- 2020年9月(9)
- 2020年8月(10)
- 2020年7月(9)
- 2020年6月(18)
- 2020年5月(4)
- 2020年4月(25)
- 2020年3月(38)
- 2020年1月(21)
- 2019年12月(13)
- 2019年11月(29)
- 2019年10月(44)
- 2019年9月(17)
- 2019年8月(18)
- 2019年7月(25)
- 2019年6月(25)
- 2019年5月(17)
- 2019年4月(10)
- 2019年3月(36)
- 2019年2月(35)
- 2019年1月(28)
- 2018年12月(30)
- 2018年11月(22)
- 2018年10月(4)
- 2018年9月(7)
- 2018年8月(13)
- 2018年7月(13)
- 2018年6月(6)
- 2018年5月(5)
- 2018年4月(13)
- 2018年3月(5)
- 2018年2月(3)
- 2018年1月(8)
- 2017年12月(35)
- 2017年11月(17)
- 2017年10月(16)
- 2017年9月(17)
- 2017年8月(20)
- 2017年7月(34)
- 2017年6月(17)
- 2017年5月(15)
- 2017年4月(32)
- 2017年3月(8)
- 2017年2月(2)
- 2017年1月(5)
- 2016年12月(14)
- 2016年11月(26)
- 2016年10月(12)
- 2016年9月(25)
- 2016年8月(32)
- 2016年7月(14)
- 2016年6月(21)
- 2016年5月(17)
- 2016年4月(13)
- 2016年3月(8)
- 2016年2月(8)
- 2016年1月(18)
- 2015年12月(13)
- 2015年11月(15)
- 2015年10月(12)
- 2015年9月(18)
- 2015年8月(21)
- 2015年7月(35)
- 2015年6月(13)
- 2015年5月(9)
- 2015年4月(4)
- 2015年3月(5)
- 2015年2月(4)
- 2015年1月(13)
- 2014年12月(7)
- 2014年11月(5)
- 2014年10月(4)
- 2014年9月(8)
- 2014年8月(16)
- 2014年7月(26)
- 2014年6月(22)
- 2014年5月(28)
- 2014年4月(15)
友情链接
- Unity官网
- Unity圣典
- Unity在线手册
- Unity中文手册(圣典)
- Unity官方中文论坛
- Unity游戏蛮牛用户文档
- Unity下载存档
- Unity引擎源码下载
- Unity服务
- Unity Ads
- wiki.unity3d
- Visual Studio Code官网
- SenseAR开发文档
- MSDN
- C# 参考
- C# 编程指南
- .NET Framework类库
- .NET 文档
- .NET 开发
- WPF官方文档
- uLua
- xLua
- SharpZipLib
- Protobuf-net
- Protobuf.js
- OpenSSL
- OPEN CASCADE
- JSON
- MessagePack
- C在线工具
- 游戏蛮牛
- GreenVPN
- 聚合数据
- 热云
- 融云
- 腾讯云
- 腾讯开放平台
- 腾讯游戏服务
- 腾讯游戏开发者平台
- 腾讯课堂
- 微信开放平台
- 腾讯实时音视频
- 腾讯即时通信IM
- 微信公众平台技术文档
- 白鹭引擎官网
- 白鹭引擎开放平台
- 白鹭引擎开发文档
- FairyGUI编辑器
- PureMVC-TypeScript
- 讯飞开放平台
- 亲加通讯云
- Cygwin
- Mono开发者联盟
- Scut游戏服务器引擎
- KBEngine游戏服务器引擎
- Photon游戏服务器引擎
- 码云
- SharpSvn
- 腾讯bugly
- 4399原创平台
- 开源中国
- Firebase
- Firebase-Admob-Unity
- google-services-unity
- Firebase SDK for Unity
- Google-Firebase-SDK
- AppsFlyer SDK
- android-repository
- CQASO
- Facebook开发者平台
- gradle下载
- GradleBuildTool下载
- Android Developers
- Google中国开发者
- AndroidDevTools
- Android社区
- Android开发工具
- Google Play Games Services
- Google商店
- Google APIs for Android
- 金钱豹VPN
- TouchSense SDK
- MakeHuman
- Online RSA Key Converter
- Windows UWP应用
- Visual Studio For Unity
- Open CASCADE Technology
- 慕课网
- 阿里云服务器ECS
- 在线免费文字转语音系统
- AI Studio
- 网云穿
- 百度网盘开放平台
- 迅捷画图
- 菜鸟工具
- [CSDN] 程序员研修院
- 华为人脸识别
- 百度AR导航导览SDK
- 海康威视官网
- 海康开放平台
- 海康SDK下载
交流QQ群
-
Flash游戏设计: 86184192
Unity游戏设计: 171855449
游戏设计订阅号
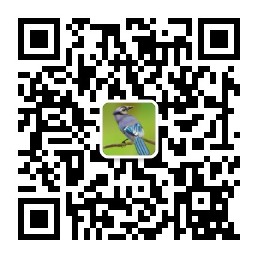