物体沿贝塞尔曲线运动
作者:追风剑情 发布于:2019-5-23 21:45 分类:Unity3d
一、新建场景,如图
TrackDraw.cs
using System.Collections; using System.Collections.Generic; using UnityEngine; ////// 在场景中显示轨迹 /// [ExecuteInEditMode] public class TrackDraw : MonoBehaviour { public bool ShowTrack = true; public LineRenderer lineRender; public int linePointCount = 51; public bool UseWorldPosition = true; public Transform[] PathTrans; private Vector3[] pathPos; private Vector3[] pathWorldPos; private Vector3[] pathLocalPos; private Vector3[] linePos; void UpdatePathData() { if (pathPos == null || pathPos.Length != PathTrans.Length) { pathPos = new Vector3[PathTrans.Length]; pathWorldPos = new Vector3[PathTrans.Length]; pathLocalPos = new Vector3[PathTrans.Length]; } for (int i = 0; i < PathTrans.Length; i++) { Vector3 pos = UseWorldPosition ? PathTrans[i].position : PathTrans[i].localPosition; pathPos[i] = pos; pathWorldPos[i] = PathTrans[i].position; pathLocalPos[i] = PathTrans[i].localPosition; } } void ShowPath() { UpdatePathData(); if (linePos == null || linePos.Length <= 0 || linePos.Length != linePointCount) linePos = new Vector3[linePointCount]; int i = 0; for (float t = 0; t <= 1; t += 0.02f) { Vector3 tp = Bezier.Lerp(pathPos, t); linePos[i++] = tp; } if (lineRender == null) { lineRender = gameObject.GetComponent(); if (lineRender == null) lineRender = gameObject.AddComponent (); } lineRender.positionCount = linePos.Length; lineRender.SetPositions(linePos); } private void OnDrawGizmos() { if (ShowTrack) ShowPath(); else lineRender.positionCount = 0; } // 使用世界坐标插值 t=[0, 1] public Vector3 Lerp(float t) { if (pathPos == null) UpdatePathData(); return Bezier.Lerp(pathPos, t); } // 使用本地坐标插值 t=[0, 1] public Vector3 LocalLerp(float t) { if (pathLocalPos == null) UpdatePathData(); return Bezier.Lerp(pathLocalPos, t); } }
TrackController.cs
using System.Collections; using System.Collections.Generic; using UnityEngine; /// <summary> /// 轨迹控制器 /// </summary> public class TrackController : MonoBehaviour { public TrackDraw trackDraw; public double speed = 0.02; public Transform[] PathTrans; private Bezier.Point[] points; private Transform mTransform; private double t = 0; void Start() { mTransform = transform; CopyTrackDrawPath(); points = new Bezier.Point[PathTrans.Length]; for (int i=0; i < PathTrans.Length; i++) { Vector3 pos = PathTrans[i].position; Bezier.Point p = new Bezier.Point(); p.x = pos.x; p.y = pos.y; p.z = pos.z; points[i] = p; } } void Update() { MoveTo(t); t += speed * Time.deltaTime; if (t > 1) t -= 1; Debug.Log(t); } void MoveTo(double t) { Bezier.Point tp = Bezier.Lerp(points, t); Vector3 pos = mTransform.position; pos.x = (float)tp.x; pos.y = (float)tp.y; pos.z = (float)tp.z; mTransform.position = pos; } [ContextMenu("Copy TrackDraw Path")] private void CopyTrackDrawPath() { if (trackDraw == null) return; PathTrans = new Transform[trackDraw.PathTrans.Length]; for (int i = 0; i < PathTrans.Length; i++) PathTrans[i] = trackDraw.PathTrans[i]; } }
Bezier.cs
using System.Collections; using System.Collections.Generic; using UnityEngine; /// <summary> /// 贝塞尔曲线 /// 参见 http://www.devacg.com/?post=912 /// </summary> public class Bezier { /// <summary> /// 作图法算法实现 /// </summary> /// <param name="P">控制点坐标</param> /// <param name="t">插值参数</param> /// <returns>返回曲线在参数t的坐标值</returns> public static Point Lerp(Point[] P, double t) { int m, i;//m 边数 int n = P.Length; //n 控制点个数 Point P0 = null; Point[] R, Q; R = new Point[n]; Q = new Point[n]; for (i = 0; i < n; i++) { R[i] = P[i];//将控制点坐标P保存于R中 Q[i] = new Point(); } //作n次外部循环, //每次循环都计算控制多边形上所有的m条边以参数t为分割比例的坐标值 for (m = n - 1; m > 0; m--) { //作m次内部循环, //每次循环计算控制多边形上一条边以参数t为分割比例的坐标值 for (i = 0; i <= m - 1; i++) { //n次Bezier曲线在点t的值,可由两条n-1次bezier曲线 //在点t的值通过线性组合而求得 Q[i].x = R[i].x + t * (R[i + 1].x - R[i].x); Q[i].y = R[i].y + t * (R[i + 1].y - R[i].y); Q[i].z = R[i].z + t * (R[i + 1].z - R[i].z); } for (i = 0; i <= m - 1; i++) R[i] = Q[i]; } P0 = R[0]; R = null; Q = null; return P0; } public class Point { public double x; public double y; public double z; } }
运行测试
标签: Unity3d
日历
最新文章
随机文章
热门文章
分类
存档
- 2024年4月(9)
- 2024年3月(11)
- 2024年2月(24)
- 2024年1月(12)
- 2023年12月(3)
- 2023年11月(9)
- 2023年10月(7)
- 2023年9月(2)
- 2023年8月(7)
- 2023年7月(9)
- 2023年6月(6)
- 2023年5月(7)
- 2023年4月(11)
- 2023年3月(6)
- 2023年2月(11)
- 2023年1月(8)
- 2022年12月(2)
- 2022年11月(4)
- 2022年10月(10)
- 2022年9月(2)
- 2022年8月(13)
- 2022年7月(7)
- 2022年6月(11)
- 2022年5月(18)
- 2022年4月(29)
- 2022年3月(5)
- 2022年2月(6)
- 2022年1月(8)
- 2021年12月(5)
- 2021年11月(3)
- 2021年10月(4)
- 2021年9月(9)
- 2021年8月(14)
- 2021年7月(8)
- 2021年6月(5)
- 2021年5月(2)
- 2021年4月(3)
- 2021年3月(7)
- 2021年2月(2)
- 2021年1月(8)
- 2020年12月(7)
- 2020年11月(2)
- 2020年10月(6)
- 2020年9月(9)
- 2020年8月(10)
- 2020年7月(9)
- 2020年6月(18)
- 2020年5月(4)
- 2020年4月(25)
- 2020年3月(38)
- 2020年1月(21)
- 2019年12月(13)
- 2019年11月(29)
- 2019年10月(44)
- 2019年9月(17)
- 2019年8月(18)
- 2019年7月(25)
- 2019年6月(25)
- 2019年5月(17)
- 2019年4月(10)
- 2019年3月(36)
- 2019年2月(35)
- 2019年1月(28)
- 2018年12月(30)
- 2018年11月(22)
- 2018年10月(4)
- 2018年9月(7)
- 2018年8月(13)
- 2018年7月(13)
- 2018年6月(6)
- 2018年5月(5)
- 2018年4月(13)
- 2018年3月(5)
- 2018年2月(3)
- 2018年1月(8)
- 2017年12月(35)
- 2017年11月(17)
- 2017年10月(16)
- 2017年9月(17)
- 2017年8月(20)
- 2017年7月(34)
- 2017年6月(17)
- 2017年5月(15)
- 2017年4月(32)
- 2017年3月(8)
- 2017年2月(2)
- 2017年1月(5)
- 2016年12月(14)
- 2016年11月(26)
- 2016年10月(12)
- 2016年9月(25)
- 2016年8月(32)
- 2016年7月(14)
- 2016年6月(21)
- 2016年5月(17)
- 2016年4月(13)
- 2016年3月(8)
- 2016年2月(8)
- 2016年1月(18)
- 2015年12月(13)
- 2015年11月(15)
- 2015年10月(12)
- 2015年9月(18)
- 2015年8月(21)
- 2015年7月(35)
- 2015年6月(13)
- 2015年5月(9)
- 2015年4月(4)
- 2015年3月(5)
- 2015年2月(4)
- 2015年1月(13)
- 2014年12月(7)
- 2014年11月(5)
- 2014年10月(4)
- 2014年9月(8)
- 2014年8月(16)
- 2014年7月(26)
- 2014年6月(22)
- 2014年5月(28)
- 2014年4月(15)
友情链接
- Unity官网
- Unity圣典
- Unity在线手册
- Unity中文手册(圣典)
- Unity官方中文论坛
- Unity游戏蛮牛用户文档
- Unity下载存档
- Unity引擎源码下载
- Unity服务
- Unity Ads
- wiki.unity3d
- Visual Studio Code官网
- SenseAR开发文档
- MSDN
- C# 参考
- C# 编程指南
- .NET Framework类库
- .NET 文档
- .NET 开发
- WPF官方文档
- uLua
- xLua
- SharpZipLib
- Protobuf-net
- Protobuf.js
- OpenSSL
- OPEN CASCADE
- JSON
- MessagePack
- C在线工具
- 游戏蛮牛
- GreenVPN
- 聚合数据
- 热云
- 融云
- 腾讯云
- 腾讯开放平台
- 腾讯游戏服务
- 腾讯游戏开发者平台
- 腾讯课堂
- 微信开放平台
- 腾讯实时音视频
- 腾讯即时通信IM
- 微信公众平台技术文档
- 白鹭引擎官网
- 白鹭引擎开放平台
- 白鹭引擎开发文档
- FairyGUI编辑器
- PureMVC-TypeScript
- 讯飞开放平台
- 亲加通讯云
- Cygwin
- Mono开发者联盟
- Scut游戏服务器引擎
- KBEngine游戏服务器引擎
- Photon游戏服务器引擎
- 码云
- SharpSvn
- 腾讯bugly
- 4399原创平台
- 开源中国
- Firebase
- Firebase-Admob-Unity
- google-services-unity
- Firebase SDK for Unity
- Google-Firebase-SDK
- AppsFlyer SDK
- android-repository
- CQASO
- Facebook开发者平台
- gradle下载
- GradleBuildTool下载
- Android Developers
- Google中国开发者
- AndroidDevTools
- Android社区
- Android开发工具
- Google Play Games Services
- Google商店
- Google APIs for Android
- 金钱豹VPN
- TouchSense SDK
- MakeHuman
- Online RSA Key Converter
- Windows UWP应用
- Visual Studio For Unity
- Open CASCADE Technology
- 慕课网
- 阿里云服务器ECS
- 在线免费文字转语音系统
- AI Studio
- 网云穿
- 百度网盘开放平台
- 迅捷画图
- 菜鸟工具
- [CSDN] 程序员研修院
- 华为人脸识别
- 百度AR导航导览SDK
- 海康威视官网
- 海康开放平台
- 海康SDK下载
交流QQ群
-
Flash游戏设计: 86184192
Unity游戏设计: 171855449
游戏设计订阅号
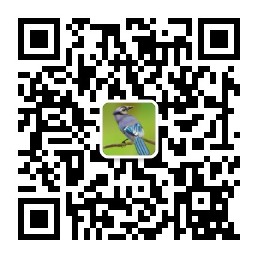