RLE压缩算法
作者:追风剑情 发布于:2016-4-16 15:11 分类:C#
RLE(Run Length Encoding)压缩算法即行程长度压缩算法,也称游程长度压缩算法,是最早出现、也是最简单的无损数据压缩算法。RLE压缩算法对黑白图像和基于调色板的单调图像有很高的压缩效率,不仅常用于处理图像数据,在传真机上也得到了广泛的应用。
压缩格式
[长度标识位] [数据块]
长度标识位通常用一个字节标识,字节最高位为1表示后面的数据块是连续重复数据。字节最高位为0表示后面的数据块是非连续数据。把最高位除开,只有剩下的7位用来表示数据长度(即最大值是127)。
重复数据: 连续出现次数2次以上。(例如: 65, 65, 65, ...)
以下是RLE算法实现代码
using System; /// <summary> /// 行程长度压缩算法 RLE(Run Length Encoding) /// 最简单的无损数据压缩算法 /// </summary> public class RLE { /// <summary> /// 压缩 /// </summary> /// <param name="inbuf">原始数据</param> /// <returns>压缩后的数据</returns> public static byte[] Encode(byte[] inbuf) { int encSize = 0; int inbufPos = 0; int inBuffSize = inbuf.Length; byte[] buff = new byte[inBuffSize]; while (inbufPos < inBuffSize) { byte count = 0; if (IsRepetitionStart(inbuf, inbufPos)) { count = GetRepetitionCount(inbuf, inbufPos); //长度字节最高位设置为1 buff[encSize++] = (byte)(count | 0x80); buff[encSize++] = inbuf[inbufPos]; inbufPos += count; } else { count = GetNonRepetitionCount(inbuf, inbufPos); buff[encSize++] = count; //逐个复制这些数据 for (int i = 0; i < count; i++) { buff[encSize++] = inbuf[inbufPos++]; } } } byte[] outbuf = new byte[encSize]; Array.Copy(buff, 0, outbuf, 0, encSize); return outbuf; } /// <summary> /// 解压 /// </summary> /// <param name="inbuf">压缩数据</param> /// <param name="outBuffSize">输出缓冲区大小</param> /// <returns>解压后的数据</returns> public static byte[] Decode(byte[] inbuf, int outBuffSize = -1) { int i; int decSize = 0; int count = 0; int inbufPos = 0; int inbufSize = inbuf.Length; if (-1 == outBuffSize) outBuffSize = inbufSize * 5; byte[] buff = new byte[outBuffSize]; while (inbufPos < inbufSize) { byte sign = inbuf[inbufPos++]; count = sign & 0x3F; //输出缓冲区空间不够了 if (decSize + count > outBuffSize) { Console.WriteLine("错误: 输出缓冲区空间不够"); return null; } if ((sign & 0x80) == 0x80)//连续重复数据标志 { for (i = 0; i < count; i++) { buff[decSize++] = inbuf[inbufPos]; } inbufPos++; } else { for (i = 0; i < count; i++) { buff[decSize++] = inbuf[inbufPos++]; } } } byte[] outbuf = new byte[decSize]; Array.Copy(buff, 0, outbuf, 0, decSize); return outbuf; } /// <summary> /// 是否连续三个字节数据相同 /// </summary> /// <returns></returns> static bool IsRepetitionStart(byte[] src, int srcLeft) { if (srcLeft + 3 >= src.Length) return false; int byte1 = src[srcLeft]; int byte2 = src[srcLeft + 1]; int byte3 = src[srcLeft + 2]; return (byte1 == byte2 && byte1 == byte3); } static byte GetRepetitionCount(byte[] src, int srcPos) { int endLeft = srcPos + 127; if (endLeft >= src.Length) endLeft = src.Length - 1; byte count = 1; for (int i = srcPos; i < endLeft; i++) { if (src[i] != src[i + 1]) break; count++; } return count; } static byte GetNonRepetitionCount(byte[] src, int srcPos) { //只剩最后两字节数据了 if (src.Length - srcPos <= 3) return 2; int endLeft = srcPos + 127; if (endLeft >= src.Length) endLeft = src.Length - 1; byte count = 0; for (int i = srcPos; i <= endLeft; i++) { if (IsRepetitionStart(src, i)) break; count++; } return count; } }
测试
using System; using System.Collections.Generic; using System.Linq; using System.Text; class Program { static void Main(string[] args) { //测试数据 byte[] data = new byte[] { 10, 20, 20, 30, 30, 30, 40, 40, 40, 40, 50, 50, 50, 50, 50, 60, 60, 60, 60, 60, 60, 70, 70, 80, 80, 90 }; Console.WriteLine("原始数据"); for (int i = 0; i < data.Length; i++) Console.Write(data[i]+", "); Console.WriteLine(); Console.WriteLine("RLE压缩数据"); byte[] en_data = RLE.Encode(data); for (int i = 0; i < en_data.Length; i++) Console.Write(en_data[i] + ", "); Console.WriteLine(); Console.WriteLine("RLE解压数据"); byte[] de_data = RLE.Decode(en_data); for (int i = 0; i < de_data.Length; i++) Console.Write(de_data[i] + ", "); Console.Read(); } }
运行效果
标签: C#
日历
最新文章
随机文章
热门文章
分类
存档
- 2024年4月(9)
- 2024年3月(11)
- 2024年2月(24)
- 2024年1月(12)
- 2023年12月(3)
- 2023年11月(9)
- 2023年10月(7)
- 2023年9月(2)
- 2023年8月(7)
- 2023年7月(9)
- 2023年6月(6)
- 2023年5月(7)
- 2023年4月(11)
- 2023年3月(6)
- 2023年2月(11)
- 2023年1月(8)
- 2022年12月(2)
- 2022年11月(4)
- 2022年10月(10)
- 2022年9月(2)
- 2022年8月(13)
- 2022年7月(7)
- 2022年6月(11)
- 2022年5月(18)
- 2022年4月(29)
- 2022年3月(5)
- 2022年2月(6)
- 2022年1月(8)
- 2021年12月(5)
- 2021年11月(3)
- 2021年10月(4)
- 2021年9月(9)
- 2021年8月(14)
- 2021年7月(8)
- 2021年6月(5)
- 2021年5月(2)
- 2021年4月(3)
- 2021年3月(7)
- 2021年2月(2)
- 2021年1月(8)
- 2020年12月(7)
- 2020年11月(2)
- 2020年10月(6)
- 2020年9月(9)
- 2020年8月(10)
- 2020年7月(9)
- 2020年6月(18)
- 2020年5月(4)
- 2020年4月(25)
- 2020年3月(38)
- 2020年1月(21)
- 2019年12月(13)
- 2019年11月(29)
- 2019年10月(44)
- 2019年9月(17)
- 2019年8月(18)
- 2019年7月(25)
- 2019年6月(25)
- 2019年5月(17)
- 2019年4月(10)
- 2019年3月(36)
- 2019年2月(35)
- 2019年1月(28)
- 2018年12月(30)
- 2018年11月(22)
- 2018年10月(4)
- 2018年9月(7)
- 2018年8月(13)
- 2018年7月(13)
- 2018年6月(6)
- 2018年5月(5)
- 2018年4月(13)
- 2018年3月(5)
- 2018年2月(3)
- 2018年1月(8)
- 2017年12月(35)
- 2017年11月(17)
- 2017年10月(16)
- 2017年9月(17)
- 2017年8月(20)
- 2017年7月(34)
- 2017年6月(17)
- 2017年5月(15)
- 2017年4月(32)
- 2017年3月(8)
- 2017年2月(2)
- 2017年1月(5)
- 2016年12月(14)
- 2016年11月(26)
- 2016年10月(12)
- 2016年9月(25)
- 2016年8月(32)
- 2016年7月(14)
- 2016年6月(21)
- 2016年5月(17)
- 2016年4月(13)
- 2016年3月(8)
- 2016年2月(8)
- 2016年1月(18)
- 2015年12月(13)
- 2015年11月(15)
- 2015年10月(12)
- 2015年9月(18)
- 2015年8月(21)
- 2015年7月(35)
- 2015年6月(13)
- 2015年5月(9)
- 2015年4月(4)
- 2015年3月(5)
- 2015年2月(4)
- 2015年1月(13)
- 2014年12月(7)
- 2014年11月(5)
- 2014年10月(4)
- 2014年9月(8)
- 2014年8月(16)
- 2014年7月(26)
- 2014年6月(22)
- 2014年5月(28)
- 2014年4月(15)
友情链接
- Unity官网
- Unity圣典
- Unity在线手册
- Unity中文手册(圣典)
- Unity官方中文论坛
- Unity游戏蛮牛用户文档
- Unity下载存档
- Unity引擎源码下载
- Unity服务
- Unity Ads
- wiki.unity3d
- Visual Studio Code官网
- SenseAR开发文档
- MSDN
- C# 参考
- C# 编程指南
- .NET Framework类库
- .NET 文档
- .NET 开发
- WPF官方文档
- uLua
- xLua
- SharpZipLib
- Protobuf-net
- Protobuf.js
- OpenSSL
- OPEN CASCADE
- JSON
- MessagePack
- C在线工具
- 游戏蛮牛
- GreenVPN
- 聚合数据
- 热云
- 融云
- 腾讯云
- 腾讯开放平台
- 腾讯游戏服务
- 腾讯游戏开发者平台
- 腾讯课堂
- 微信开放平台
- 腾讯实时音视频
- 腾讯即时通信IM
- 微信公众平台技术文档
- 白鹭引擎官网
- 白鹭引擎开放平台
- 白鹭引擎开发文档
- FairyGUI编辑器
- PureMVC-TypeScript
- 讯飞开放平台
- 亲加通讯云
- Cygwin
- Mono开发者联盟
- Scut游戏服务器引擎
- KBEngine游戏服务器引擎
- Photon游戏服务器引擎
- 码云
- SharpSvn
- 腾讯bugly
- 4399原创平台
- 开源中国
- Firebase
- Firebase-Admob-Unity
- google-services-unity
- Firebase SDK for Unity
- Google-Firebase-SDK
- AppsFlyer SDK
- android-repository
- CQASO
- Facebook开发者平台
- gradle下载
- GradleBuildTool下载
- Android Developers
- Google中国开发者
- AndroidDevTools
- Android社区
- Android开发工具
- Google Play Games Services
- Google商店
- Google APIs for Android
- 金钱豹VPN
- TouchSense SDK
- MakeHuman
- Online RSA Key Converter
- Windows UWP应用
- Visual Studio For Unity
- Open CASCADE Technology
- 慕课网
- 阿里云服务器ECS
- 在线免费文字转语音系统
- AI Studio
- 网云穿
- 百度网盘开放平台
- 迅捷画图
- 菜鸟工具
- [CSDN] 程序员研修院
- 华为人脸识别
- 百度AR导航导览SDK
- 海康威视官网
- 海康开放平台
- 海康SDK下载
交流QQ群
-
Flash游戏设计: 86184192
Unity游戏设计: 171855449
游戏设计订阅号
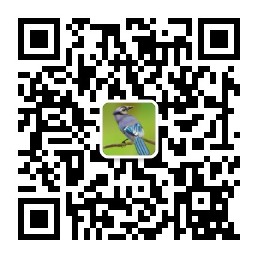