贴图九宫缩放
作者:追风剑情 发布于:2023-4-13 17:43 分类:Unity3d
1、创建九宫缩放Shader
//用于Quad Mesh贴图 九宫缩放 Shader "Unlit/Transparent (9Palace Zoom)" { Properties { [NoScaleOffset] _MainTex ("Base (RGB) Trans (A)", 2D) = "white" {} //左边距 _L("Border L", Range(0, 0.5)) = 0.2 //右边距 _R("Border R", Range(0, 0.5)) = 0.2 //上边距 _T("Border T", Range(0, 0.5)) = 0.2 //下边距 _B("Border B", Range(0, 0.5)) = 0.2 //针对四个角的缩放值 _ScaleX("Scale X", Float) = 1 _ScaleY("Scale Y", Float) = 1 } SubShader { Tags {"Queue"="Transparent" "IgnoreProjector"="True" "RenderType"="Transparent"} LOD 100 ZWrite Off Blend SrcAlpha OneMinusSrcAlpha Pass { CGPROGRAM #pragma vertex vert #pragma fragment frag #pragma target 2.0 #pragma multi_compile_fog #include "UnityCG.cginc" struct appdata_t { float4 vertex : POSITION; float2 texcoord : TEXCOORD0; UNITY_VERTEX_INPUT_INSTANCE_ID }; struct v2f { float4 vertex : SV_POSITION; float2 texcoord : TEXCOORD0; UNITY_FOG_COORDS(1) UNITY_VERTEX_OUTPUT_STEREO }; sampler2D _MainTex; float4 _MainTex_ST; fixed _L; fixed _R; fixed _T; fixed _B; fixed _ScaleX; fixed _ScaleY; v2f vert (appdata_t v) { v2f o; UNITY_SETUP_INSTANCE_ID(v); UNITY_INITIALIZE_VERTEX_OUTPUT_STEREO(o); o.vertex = UnityObjectToClipPos(v.vertex); o.texcoord = TRANSFORM_TEX(v.texcoord, _MainTex); UNITY_TRANSFER_FOG(o,o.vertex); return o; } fixed4 frag (v2f i) : SV_Target { fixed2 uv = i.texcoord; //将原来的Border尺寸进行缩放 fixed _sL = _L * _ScaleX; fixed _sR = _R * _ScaleX; fixed _sT = _T * _ScaleY; fixed _sB = _B * _ScaleY; //通过插值算法将四个角的画面进行缩放 //插值比例 fixed _wL = uv.x / _sL; fixed _wR = (uv.x - (1 - _sR)) / _sR; fixed _wT = uv.y / _sT; fixed _wB = (uv.y - (1 - _sB)) / _sB; //对x坐标插值 /* 普通算法 if (uv.x < _sL) // step(uv.x, _sL); uv.x = lerp(0, _L, _wL); else if (uv.x > 1 - _sR) //step(1-_sR, uv.x) uv.x = lerp(1 - _R, 1, _wR); else uv.x = 0.5; //step(_sL, uv.x) * step(uv.x, 1-_sR); */ //优化算法 uv.x = lerp(0, _L, _wL) * step(uv.x, _sL) + lerp(1 - _R, 1, _wR) * step(1 - _sR, uv.x) + 0.5 * step(_sL, uv.x) * step(uv.x, 1 - _sR); //对y坐标插值 /* 普通算法 if (uv.y < _sT) uv.y = lerp(0, _T, _wT); else if (uv.y > 1 - _sT) uv.y = lerp(1 - _B, 1, _wB); else uv.y = 0.5; */ //优化算法 uv.y = lerp(0, _T, _wT) * step(uv.y, _sT) + lerp(1 - _B, 1, _wB) * step(1 - _sB, uv.y) + 0.5 * step(_sT, uv.y) * step(uv.y, 1 - _sB); //End fixed4 col = tex2D(_MainTex, uv); UNITY_APPLY_FOG(i.fogCoord, col); return col; } ENDCG } } }
2、创建九宫缩放脚本(非必须)
using UnityEngine; [ExecuteInEditMode] public class Transparent9PalaceZoom : MonoBehaviour { [SerializeField] private MeshRenderer meshRenderer; [SerializeField] private Texture2D mainTexture; [SerializeField] private float borderL = 0.2f; [SerializeField] private float borderR = 0.2f; [SerializeField] private float borderT = 0.2f; [SerializeField] private float borderB = 0.2f; [SerializeField] private float scaleX = 1; [SerializeField] private float scaleY = 1; [SerializeField] private bool m_Update = false; private void Update() { if (!m_Update) return; m_Update = false; //更新材质属性值 if (meshRenderer == null) meshRenderer = this.GetComponent<MeshRenderer>(); if (meshRenderer == null) return; MaterialPropertyBlock properties = new MaterialPropertyBlock(); if (mainTexture != null) properties.SetTexture("_MainTex", mainTexture); properties.SetFloat("_L", borderL); properties.SetFloat("_R", borderR); properties.SetFloat("_T", borderT); properties.SetFloat("_B", borderB); properties.SetFloat("_ScaleX", scaleX); properties.SetFloat("_ScaleY", scaleY); meshRenderer.SetPropertyBlock(properties); } }
3、创建九宫缩放Material
4、创建3个Quad
给每个Quad设置不同的九宫缩放参数
显示效果
日历
最新文章
随机文章
热门文章
分类
存档
- 2024年7月(11)
- 2024年6月(3)
- 2024年5月(9)
- 2024年4月(10)
- 2024年3月(11)
- 2024年2月(24)
- 2024年1月(12)
- 2023年12月(3)
- 2023年11月(9)
- 2023年10月(7)
- 2023年9月(2)
- 2023年8月(7)
- 2023年7月(9)
- 2023年6月(6)
- 2023年5月(7)
- 2023年4月(11)
- 2023年3月(6)
- 2023年2月(11)
- 2023年1月(8)
- 2022年12月(2)
- 2022年11月(4)
- 2022年10月(10)
- 2022年9月(2)
- 2022年8月(13)
- 2022年7月(7)
- 2022年6月(11)
- 2022年5月(18)
- 2022年4月(29)
- 2022年3月(5)
- 2022年2月(6)
- 2022年1月(8)
- 2021年12月(5)
- 2021年11月(3)
- 2021年10月(4)
- 2021年9月(9)
- 2021年8月(14)
- 2021年7月(8)
- 2021年6月(5)
- 2021年5月(2)
- 2021年4月(3)
- 2021年3月(7)
- 2021年2月(2)
- 2021年1月(8)
- 2020年12月(7)
- 2020年11月(2)
- 2020年10月(6)
- 2020年9月(9)
- 2020年8月(10)
- 2020年7月(9)
- 2020年6月(18)
- 2020年5月(4)
- 2020年4月(25)
- 2020年3月(38)
- 2020年1月(21)
- 2019年12月(13)
- 2019年11月(29)
- 2019年10月(44)
- 2019年9月(17)
- 2019年8月(18)
- 2019年7月(25)
- 2019年6月(25)
- 2019年5月(17)
- 2019年4月(10)
- 2019年3月(36)
- 2019年2月(35)
- 2019年1月(28)
- 2018年12月(30)
- 2018年11月(22)
- 2018年10月(4)
- 2018年9月(7)
- 2018年8月(13)
- 2018年7月(13)
- 2018年6月(6)
- 2018年5月(5)
- 2018年4月(13)
- 2018年3月(5)
- 2018年2月(3)
- 2018年1月(8)
- 2017年12月(35)
- 2017年11月(17)
- 2017年10月(16)
- 2017年9月(17)
- 2017年8月(20)
- 2017年7月(34)
- 2017年6月(17)
- 2017年5月(15)
- 2017年4月(32)
- 2017年3月(8)
- 2017年2月(2)
- 2017年1月(5)
- 2016年12月(14)
- 2016年11月(26)
- 2016年10月(12)
- 2016年9月(25)
- 2016年8月(32)
- 2016年7月(14)
- 2016年6月(21)
- 2016年5月(17)
- 2016年4月(13)
- 2016年3月(8)
- 2016年2月(8)
- 2016年1月(18)
- 2015年12月(13)
- 2015年11月(15)
- 2015年10月(12)
- 2015年9月(18)
- 2015年8月(21)
- 2015年7月(35)
- 2015年6月(13)
- 2015年5月(9)
- 2015年4月(4)
- 2015年3月(5)
- 2015年2月(4)
- 2015年1月(13)
- 2014年12月(7)
- 2014年11月(5)
- 2014年10月(4)
- 2014年9月(8)
- 2014年8月(16)
- 2014年7月(26)
- 2014年6月(22)
- 2014年5月(28)
- 2014年4月(15)
友情链接
- Unity官网
- Unity圣典
- Unity在线手册
- Unity中文手册(圣典)
- Unity官方中文论坛
- Unity游戏蛮牛用户文档
- Unity下载存档
- Unity引擎源码下载
- Unity服务
- Unity Ads
- wiki.unity3d
- Visual Studio Code官网
- SenseAR开发文档
- MSDN
- C# 参考
- C# 编程指南
- .NET Framework类库
- .NET 文档
- .NET 开发
- WPF官方文档
- uLua
- xLua
- SharpZipLib
- Protobuf-net
- Protobuf.js
- OpenSSL
- OPEN CASCADE
- JSON
- MessagePack
- C在线工具
- 游戏蛮牛
- GreenVPN
- 聚合数据
- 热云
- 融云
- 腾讯云
- 腾讯开放平台
- 腾讯游戏服务
- 腾讯游戏开发者平台
- 腾讯课堂
- 微信开放平台
- 腾讯实时音视频
- 腾讯即时通信IM
- 微信公众平台技术文档
- 白鹭引擎官网
- 白鹭引擎开放平台
- 白鹭引擎开发文档
- FairyGUI编辑器
- PureMVC-TypeScript
- 讯飞开放平台
- 亲加通讯云
- Cygwin
- Mono开发者联盟
- Scut游戏服务器引擎
- KBEngine游戏服务器引擎
- Photon游戏服务器引擎
- 码云
- SharpSvn
- 腾讯bugly
- 4399原创平台
- 开源中国
- Firebase
- Firebase-Admob-Unity
- google-services-unity
- Firebase SDK for Unity
- Google-Firebase-SDK
- AppsFlyer SDK
- android-repository
- CQASO
- Facebook开发者平台
- gradle下载
- GradleBuildTool下载
- Android Developers
- Google中国开发者
- AndroidDevTools
- Android社区
- Android开发工具
- Google Play Games Services
- Google商店
- Google APIs for Android
- 金钱豹VPN
- TouchSense SDK
- MakeHuman
- Online RSA Key Converter
- Windows UWP应用
- Visual Studio For Unity
- Open CASCADE Technology
- 慕课网
- 阿里云服务器ECS
- 在线免费文字转语音系统
- AI Studio
- 网云穿
- 百度网盘开放平台
- 迅捷画图
- 菜鸟工具
- [CSDN] 程序员研修院
- 华为人脸识别
- 百度AR导航导览SDK
- 海康威视官网
- 海康开放平台
- 海康SDK下载
交流QQ群
-
Flash游戏设计: 86184192
Unity游戏设计: 171855449
游戏设计订阅号
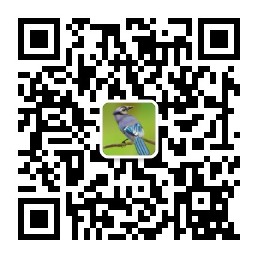