Linux下编译C语言
作者:追风剑情 发布于:2020-7-2 18:12 分类:C
目录规范
参考 https://www.cnblogs.com/aidata/p/11753179.html
/usr相当于C:/Windows
/usr/lib相当于C:/Windows/System32
/usr/local相当于C:/Program Files/
/opt相当于D:/Software
/usr/src系统级源码目录
/usr/local/src用户级源码目录
一、准备测试代码
testlib.h
//避免重复导入 #ifndef _TESTLIB_H_ #define _TESTLIB_H_ //平台判断 #if(defined _WIN64 || defined _WIN32) __declspec(dllexport) char* helloWorld(); #elif __linux__ char* helloWorld(); #endif #endif
testlib.c
#if(defined _WIN64 || defined _WIN32) #define _CRT_SECURE_NO_WARNINGS #endif #include <string.h> #include "testlib.h" char str[12]; char * helloWorld() { strcpy(str, "Hello World"); return str; }
二、编译.so
以下是Xshell截图 gcc xxx.c -fPIC -shared -o libxxx.so
Windows下使用NDK编译不同平台的.so
1、下载android-ndk-r21b-windows-x86_64.zip
2、创建源代码目录
注意:jni是ndk-build命令默认搜索的目录,将代码文件、Android.mk、Application.mk放到jni目录下。
LOCAL_PATH := $(call my-dir) include $(CLEAR_VARS) LOCAL_MODULE := libtest LOCAL_LDFLAGS := -Wl,--build-id LOCAL_SRC_FILES := testlib.c include $(BUILD_SHARED_LIBRARY)
APP_ABI := armeabi-v7a arm64-v8a x86
3、cd到src目录运行ndk-build命令
看到以上输出说明对应CPU架构的.so文件已经生成
在Linux下使用ndk-build编译不同平台的.so
以下都为Xshell截图
使用ndk-build命令编译
build.sh
#!/bin/bash PWD=$(pwd) NDK=~/opt/android-ndk-r21b BUILDER=${NDK}/ndk-build ABS=${PWD}/jni/Android.mk NAM=${PWD}/jni/Application.mk ${BUILDER} NDK_PROJECT_PATH=${PWD} APP_BUILD_SCRIPT=${ABS} NDK_APPLICATION_MK=${NAM}
利用shell脚本编译如果报下面的错
-bash: ./build.sh: /bin/bash^M: 坏的解释器: 没有那个文件或目录
原因
是因为在Windows中编辑的文件换行符造成的,将全部\r\n替换成\n即可。
解决方法
用vim打开,vim build.sh
然后执行
:set ff=unix
:wq
运行脚本前需要先开权限
chmod +x build.sh
./build.sh
使用linux的zip命令将生成的libs目录压缩成zip后,再利用sz命令取回到windows中使用
在Unity中调用.so接口
编写C#测试代码
using System; using System.Collections; using System.Collections.Generic; using System.Text; using System.Runtime.InteropServices; using UnityEngine; using UnityEngine.UI; public class DllWrap : MonoBehaviour { //调用本地代码官方文档 //https://docs.unity3d.com/cn/current/Manual/NativePlugins.html #if UNITY_IPHONE && !UNITY_EDITOR [DllImport ("__Internal")] #else [DllImport("test")] #endif private static extern IntPtr helloWorld(); public Text text; void Start() { Debug.Log("Call so interface. helloWorld()"); int len = 64; //c返回字符串指针时c#要用结构体指针接收并通过新建一个托管内存拷贝 //参考 https://www.cnblogs.com/huangchaoqun/p/7599939.html IntPtr str = helloWorld(); string s = Marshal.PtrToStringAnsi(str, len); if (s == null) { byte[] buffer = new byte[len]; Marshal.Copy(str, buffer, 0, len); s = Encoding.UTF8.GetString(buffer); } Debug.LogFormat("s={0}", s); text.text = s; } }
标签: C语言
日历
最新文章
随机文章
热门文章
分类
存档
- 2024年4月(10)
- 2024年3月(11)
- 2024年2月(24)
- 2024年1月(12)
- 2023年12月(3)
- 2023年11月(9)
- 2023年10月(7)
- 2023年9月(2)
- 2023年8月(7)
- 2023年7月(9)
- 2023年6月(6)
- 2023年5月(7)
- 2023年4月(11)
- 2023年3月(6)
- 2023年2月(11)
- 2023年1月(8)
- 2022年12月(2)
- 2022年11月(4)
- 2022年10月(10)
- 2022年9月(2)
- 2022年8月(13)
- 2022年7月(7)
- 2022年6月(11)
- 2022年5月(18)
- 2022年4月(29)
- 2022年3月(5)
- 2022年2月(6)
- 2022年1月(8)
- 2021年12月(5)
- 2021年11月(3)
- 2021年10月(4)
- 2021年9月(9)
- 2021年8月(14)
- 2021年7月(8)
- 2021年6月(5)
- 2021年5月(2)
- 2021年4月(3)
- 2021年3月(7)
- 2021年2月(2)
- 2021年1月(8)
- 2020年12月(7)
- 2020年11月(2)
- 2020年10月(6)
- 2020年9月(9)
- 2020年8月(10)
- 2020年7月(9)
- 2020年6月(18)
- 2020年5月(4)
- 2020年4月(25)
- 2020年3月(38)
- 2020年1月(21)
- 2019年12月(13)
- 2019年11月(29)
- 2019年10月(44)
- 2019年9月(17)
- 2019年8月(18)
- 2019年7月(25)
- 2019年6月(25)
- 2019年5月(17)
- 2019年4月(10)
- 2019年3月(36)
- 2019年2月(35)
- 2019年1月(28)
- 2018年12月(30)
- 2018年11月(22)
- 2018年10月(4)
- 2018年9月(7)
- 2018年8月(13)
- 2018年7月(13)
- 2018年6月(6)
- 2018年5月(5)
- 2018年4月(13)
- 2018年3月(5)
- 2018年2月(3)
- 2018年1月(8)
- 2017年12月(35)
- 2017年11月(17)
- 2017年10月(16)
- 2017年9月(17)
- 2017年8月(20)
- 2017年7月(34)
- 2017年6月(17)
- 2017年5月(15)
- 2017年4月(32)
- 2017年3月(8)
- 2017年2月(2)
- 2017年1月(5)
- 2016年12月(14)
- 2016年11月(26)
- 2016年10月(12)
- 2016年9月(25)
- 2016年8月(32)
- 2016年7月(14)
- 2016年6月(21)
- 2016年5月(17)
- 2016年4月(13)
- 2016年3月(8)
- 2016年2月(8)
- 2016年1月(18)
- 2015年12月(13)
- 2015年11月(15)
- 2015年10月(12)
- 2015年9月(18)
- 2015年8月(21)
- 2015年7月(35)
- 2015年6月(13)
- 2015年5月(9)
- 2015年4月(4)
- 2015年3月(5)
- 2015年2月(4)
- 2015年1月(13)
- 2014年12月(7)
- 2014年11月(5)
- 2014年10月(4)
- 2014年9月(8)
- 2014年8月(16)
- 2014年7月(26)
- 2014年6月(22)
- 2014年5月(28)
- 2014年4月(15)
友情链接
- Unity官网
- Unity圣典
- Unity在线手册
- Unity中文手册(圣典)
- Unity官方中文论坛
- Unity游戏蛮牛用户文档
- Unity下载存档
- Unity引擎源码下载
- Unity服务
- Unity Ads
- wiki.unity3d
- Visual Studio Code官网
- SenseAR开发文档
- MSDN
- C# 参考
- C# 编程指南
- .NET Framework类库
- .NET 文档
- .NET 开发
- WPF官方文档
- uLua
- xLua
- SharpZipLib
- Protobuf-net
- Protobuf.js
- OpenSSL
- OPEN CASCADE
- JSON
- MessagePack
- C在线工具
- 游戏蛮牛
- GreenVPN
- 聚合数据
- 热云
- 融云
- 腾讯云
- 腾讯开放平台
- 腾讯游戏服务
- 腾讯游戏开发者平台
- 腾讯课堂
- 微信开放平台
- 腾讯实时音视频
- 腾讯即时通信IM
- 微信公众平台技术文档
- 白鹭引擎官网
- 白鹭引擎开放平台
- 白鹭引擎开发文档
- FairyGUI编辑器
- PureMVC-TypeScript
- 讯飞开放平台
- 亲加通讯云
- Cygwin
- Mono开发者联盟
- Scut游戏服务器引擎
- KBEngine游戏服务器引擎
- Photon游戏服务器引擎
- 码云
- SharpSvn
- 腾讯bugly
- 4399原创平台
- 开源中国
- Firebase
- Firebase-Admob-Unity
- google-services-unity
- Firebase SDK for Unity
- Google-Firebase-SDK
- AppsFlyer SDK
- android-repository
- CQASO
- Facebook开发者平台
- gradle下载
- GradleBuildTool下载
- Android Developers
- Google中国开发者
- AndroidDevTools
- Android社区
- Android开发工具
- Google Play Games Services
- Google商店
- Google APIs for Android
- 金钱豹VPN
- TouchSense SDK
- MakeHuman
- Online RSA Key Converter
- Windows UWP应用
- Visual Studio For Unity
- Open CASCADE Technology
- 慕课网
- 阿里云服务器ECS
- 在线免费文字转语音系统
- AI Studio
- 网云穿
- 百度网盘开放平台
- 迅捷画图
- 菜鸟工具
- [CSDN] 程序员研修院
- 华为人脸识别
- 百度AR导航导览SDK
- 海康威视官网
- 海康开放平台
- 海康SDK下载
交流QQ群
-
Flash游戏设计: 86184192
Unity游戏设计: 171855449
游戏设计订阅号
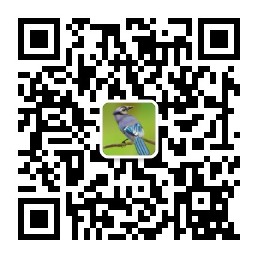